Checkout migration guide
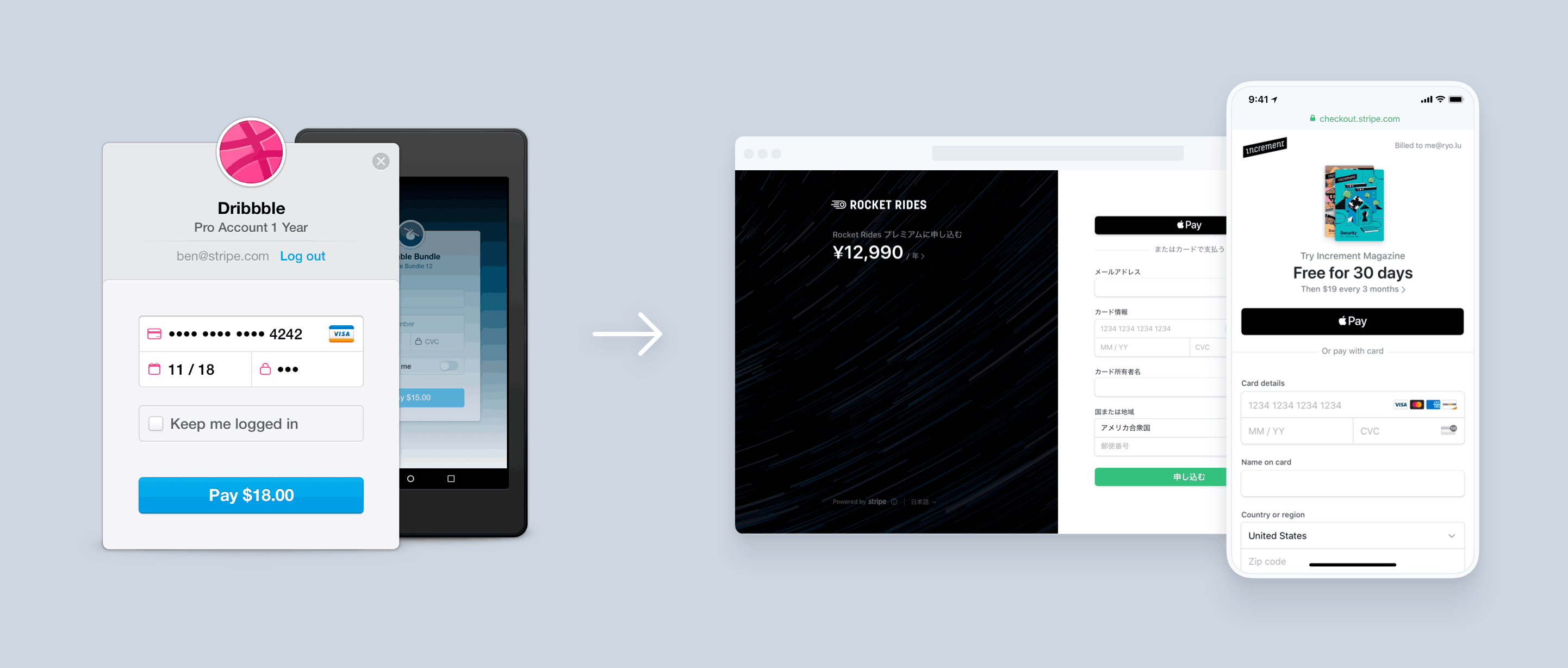
The legacy version of Checkout presented customers with a modal dialog that collected card information, and returned a token or a source to your website. In contrast, the current version of Checkout is a smart payment page hosted by Stripe that creates payments or subscriptions. It supports Apple Pay, Dynamic 3D Secure, and many other features.
The current version of Checkout provides more flexibility, with support for dynamic line items, Connect, re-using existing Customers, and advanced subscription features. You can also use the client-only integration if you want to get started more quickly or if you have a simpler product catalog.
To migrate from the legacy version to the current version, follow the guide that most closely represents your business model. Each guide recommends an integration path along with example code.
Dynamic product catalog and pricing
If you have a large product catalog or require support for dynamically generated line items (such as donations or taxes).
If you’re a SaaS provider billing users and need support for advanced features.
Connect platforms and marketplaces
If you’re operating a marketplace connecting service providers with customers.
Saving payment methods for future use
If you’re operating a business which doesn’t charge the customer until after services rendered.
Simple product catalog with fixed pricing
If you’re selling a few products with pre-determined prices.
If you’re a SaaS provider with a monthly subscription plan.
As you follow the relevant migration guide, you can also reference the conversion table for a mapping of specific parameters and configuration options between the two versions of Checkout.
Dynamic product catalog and pricing 
If you’re selling products where the amount or line items are determined dynamically (say, with a large product catalog or for donations), see accepting one-time payments.
You may have used the legacy version of Checkout to create a token or source on the client, and passed it to your server to create a charge. The current version of the Checkout server integration reverses this flow—you create a Session on your server, pass its ID to your client, redirect your customer to Checkout, who then gets redirected back to your application upon success.
Before 
With the legacy version of Checkout, you’d display the dynamic amount and description and collect card information from your customer.
<form action="/purchase" method="POST"> <script src="https://checkout.stripe.com/checkout.js" class="stripe-button" data-key=
data-name="Custom t-shirt" data-description="Your custom designed t-shirt" data-amount="{{ORDER_AMOUNT}}" data-currency="usd"> </script> </form>"pk_test_TYooMQauvdEDq54NiTphI7jx"
Next, you’d send the resulting token or source to your server and charge it.
After 
With the current version of Checkout, first create a Checkout Session on your server.
Note
If you use one of our Client libraries, upgrade to the latest version of the library in order to use the Checkout Sessions API.
Next, pass the Session ID to your client and redirect your customer to Checkout to complete payment.
const stripe = Stripe(
); const checkoutButton = document.getElementById('checkout-button'); checkoutButton.addEventListener('click', () => { stripe.redirectToCheckout({ // Make the id field from the Checkout Session creation API response // available to this file, so you can provide it as argument here // instead of the {{CHECKOUT_SESSION_ID}} placeholder. sessionId: '{{CHECKOUT_SESSION_ID}}' }) // If `redirectToCheckout` fails due to a browser or network // error, display the localized error message to your customer // using `error.message`. });'pk_test_TYooMQauvdEDq54NiTphI7jx'
The customer is redirected to the success_url
after they complete payment.
If you need to fulfill purchased goods after the payment, refer to Checkout Purchase Fulfillment.
Dynamic subscriptions 
If you’re providing subscription services that are dynamically determined or require support for other advanced features, see setting up a subscription.
You may have used the legacy version of Checkout to create a token or source on the client, and passed it to your server to create a customer and subscription. The current version of the Checkout server integration reverses this flow—you first create a Session on your server, pass its ID to your client, redirect your customer to Checkout, who then gets redirected back to your application upon success.
Before 
With the legacy version of Checkout, you’d display the subscription information and collect card information from your customer.
<form action="/subscribe" method="POST"> <script src="https://checkout.stripe.com/checkout.js" class="stripe-button" data-key=
data-name="Gold Tier" data-description="Monthly subscription with 30 days trial" data-amount="2000" data-label="Subscribe"> </script> </form>"pk_test_TYooMQauvdEDq54NiTphI7jx"
Next, you’d send the resulting token or source to your server to create a customer and a subscription.
After 
With the current version of Checkout, first create a Checkout Session on your server.
Note
If you use one of our Client libraries, upgrade to the latest version of the library in order to use the Checkout Sessions API.
Next, pass the Session ID to your client and redirect your customer to Checkout to complete payment.
const stripe = Stripe(
); const checkoutButton = document.getElementById('checkout-button'); checkoutButton.addEventListener('click', () => { stripe.redirectToCheckout({ // Make the id field from the Checkout Session creation API response // available to this file, so you can provide it as argument here // instead of the {{CHECKOUT_SESSION_ID}} placeholder. sessionId: '{{CHECKOUT_SESSION_ID}}' }) // If `redirectToCheckout` fails due to a browser or network // error, display the localized error message to your customer // using `error.message`. });'pk_test_TYooMQauvdEDq54NiTphI7jx'
The customer is redirected to the success_url
after the customer and subscription have been created.
If you need to fulfill purchased services after the payment, refer to Checkout Purchase Fulfillment.
You can also update subscription information using Checkout.
Connect platforms and marketplaces 
If you’re operating a Connect platform or marketplace and create payments involving connected accounts, consider using the current version of the Checkout server integration. Follow the instructions in the Connect guide to migrate your integration.
The following example demonstrates using the Checkout Sessions API to process a direct charge. Follow the Connect guide for details on how to create destination charges.
Before 
With the legacy version of Checkout, you would collect card information from your customer on the client.
<form action="/purchase" method="POST"> <script src="https://checkout.stripe.com/checkout.js" class="stripe-button" data-key=
data-name="Food Marketplace" data-description="10 cucumbers from Roger's Farm" data-amount="2000"> </script> </form>"pk_test_TYooMQauvdEDq54NiTphI7jx"
Next, you’d send the resulting token or source to your server and charge it on behalf of the connected account.
After 
With the current version of Checkout, first create a Checkout Session on your server on behalf of the connected account.
Note
If you use one of our Client libraries, upgrade to the latest version of the library to use the Checkout Sessions API.
Next, pass the Session ID to your client and redirect your customer to Checkout to complete payment. Make sure to provide the connected account’s ID when initializing Stripe.js.
// Initialize Stripe.js with the same connected account ID used when creating // the Checkout Session. const stripe = Stripe(
, { stripeAccount:'pk_test_TYooMQauvdEDq54NiTphI7jx'}); const checkoutButton = document.getElementById('checkout-button'); checkoutButton.addEventListener('click', () => { stripe.redirectToCheckout({ // Make the id field from the Checkout Session creation API response // available to this file, so you can provide it as argument here // instead of the {{CHECKOUT_SESSION_ID}} placeholder. sessionId: '{{CHECKOUT_SESSION_ID}}' }) // If `redirectToCheckout` fails due to a browser or network // error, display the localized error message to your customer // using `error.message`. });'{{CONNECTED_ACCOUNT_ID}}'
The customer is redirected to the success_url
after they complete payment.
If you need to fulfill purchased goods or services after the payment, refer to Checkout Purchase Fulfillment.
Saving payment methods for future use 
If you’re providing services that don’t charge your customers immediately, see setting up future payments.
You may have used the legacy version of Checkout to create a token or source on the client, and passed it to your server to save for later use. The current version of the Checkout server integration reverses this flow—you first create a Session on your server, pass its ID to your client, redirect your customer to Checkout, who then gets redirected back to your application upon success.
Before 
With the legacy version of Checkout, you’d display the charge information and collect card information from your customer.
<form action="/subscribe" method="POST"> <script src="https://checkout.stripe.com/checkout.js" class="stripe-button" data-key=
data-name="Cleaning Service" data-description="Charged after your home is spotless" data-amount="2000"> </script> </form>"pk_test_TYooMQauvdEDq54NiTphI7jx"
Next, you would send the resulting token or source to your server to eventually create a charge.
After 
With the current version of Checkout, first create a Checkout Session on your server using setup mode.
Note
If you use one of our Client libraries, upgrade to the latest version of the library to use the Checkout Sessions API.
Next, pass the Session ID to your client and redirect your customer to Checkout to gather payment method details.
const stripe = Stripe(
); const checkoutButton = document.getElementById('checkout-button'); checkoutButton.addEventListener('click', () => { stripe.redirectToCheckout({ // Make the id field from the Checkout Session creation API response // available to this file, so you can provide it as argument here // instead of the {{CHECKOUT_SESSION_ID}} placeholder. sessionId: '{{CHECKOUT_SESSION_ID}}' }) // If `redirectToCheckout` fails due to a browser or network // error, display the localized error message to your customer // using `error.message`. });'pk_test_TYooMQauvdEDq54NiTphI7jx'
The customer is redirected to the success_url
after they complete the flow.
From there, you can retrieve the Setup Intent from the Checkout flow and use it to prepare your transaction.
Simple product catalog with fixed pricing 
If you’re selling products with fixed pricing (such as t-shirts or e-books), see the guide on one-time payments to learn how to generate a code snippet to add to your website.
You may have used the legacy version of Checkout to create a token or source on the client, and passed it to your server to create a charge. The current version of Checkout automatically creates the payment for you, and no server integration is required.
Client-side code 
Server-side code 
The conversion table below provides a mapping of configuration options between the two versions of Checkout. For a full list of configuration options for the current version, see the redirectToCheckout
reference.
Simple subscriptions 
If you’re providing a simple subscription service (such as monthly access to software), see the guide on subscriptions to learn how to create a plan in the Dashboard and generate a code snippet to add to your website.
You may have used the legacy version of Checkout to create a token or source on the client, and passed it to your server to create a customer and a subscription. The current version of Checkout, however, automatically creates both the customer and the subscription for you, and no server integration is required.
Client-side code 
Server-side code 
The conversion table below provides a mapping of configuration options between the two versions of Checkout. For a full list of configuration options for the current version, see the redirectToCheckout
reference.
Parameter conversion 
The current version of Checkout supports most of the functionality of the legacy version of Checkout. However, the two versions do not share the same API. The table below maps parameters and configuration options between the legacy version and the current version.
For a full list of configuration options accepted by the current version of Checkout, see the Stripe.js reference and the API reference for Checkout Sessions.
Legacy version | Current version | Integration tips |
---|---|---|
allowRememberMe |
| The current version of Checkout doesn’t support Remember Me. To reuse existing customers, we recommend specifying the customer parameter when creating a Checkout Session. |
amount |
| The total amount is the sum of the line items you pass to Checkout. |
billingAddress |
| Checkout automatically collects the billing address when required for fraud-prevention or regulatory purposes. Set this parameter to required to always collect the billing address. |
billingAddress |
| Checkout automatically collects the billing address when required for fraud-prevention or regulatory purposes. Set this parameter to required to always collect the billing address. |
closed |
| When a customer wants to close Checkout, they either close the browser tab or navigate to the cancelUrl . |
currency |
| |
description |
| If you specify a price, Checkout displays an automatically computed description of how often payments occur. If you specify Session.line_items , Checkout displays the name for each line item. |
email |
| If you already know your customer’s email, specify it here so they do not need to enter it again. |
image |
| Checkout uses specific images for your business’s branding and for the products you’re selling. Checkout displays your business logo by default and falls back to your business icon alongside your business name. |
key |
| |
locale |
| You can specify a supported locale when creating a Checkout Session. |
name |
| If you specify a price, Checkout displays the name of the product that belongs to the price. If you specify Session.line_items , Checkout displays the name for each line item. |
panelLabel |
| Checkout automatically customizes the button text depending on the items you’re selling. For one-time payments, use submit_type to customize the button text. |
shippingAddress |
| Collect shipping address information by passing an array of allowed_countries that you want to ship to. |
token or source |
| There is no longer a callback in JavaScript when the payment completes. As your customer is paying on a different page, set the successUrl to redirect your customer after they’ve completed payment. |
zipCode |
| Checkout automatically collects the postal code when required for fraud-prevention or regulatory purposes. |