Stripe-hosted page
Explore a full, working code sample of an integration with Stripe Checkout where customers click a button on your site and get redirected to a payment page hosted by Stripe. The example includes client- and server-side code, and the payment page is prebuilt.
Install the Stripe Ruby library
Install the Stripe ruby gem and require it in your code. Alternatively, if you’re starting from scratch and need a Gemfile, download the project files using the link in the code editor.
Create a Checkout Session
Add an endpoint on your server that creates a Checkout Session. A Checkout Session controls what your customer sees on the payment page such as line items, the order amount and currency, and acceptable payment methods. We enable cards and other common payment methods for you by default, and you can enable or disable payment methods directly in the Stripe Dashboard.
Define a product to sell
Always keep sensitive information about your product inventory, such as price and availability, on your server to prevent customer manipulation from the client. Define product information when you create the Checkout Session using predefined price IDs or on the fly with price_data.
Choose a mode
To handle different transaction types, adjust the mode
parameter. For one-time payments, use payment
. To initiate recurring payments with subscriptions, switch the mode
to subscription
. And for setting up future payments, set the mode
to setup
.
Supply success and cancel URLs
Specify URLs for success and cancel pages—make sure they’re publicly accessible so Stripe can redirect customers to them. You can also handle both the success and canceled states with the same URL.
Redirect to Checkout
After creating the session, redirect your customer to the URL for the Checkout page returned in the response.
Add an order preview page
Add a page to show a preview of the customer’s order. Allow them to review or modify their order—as soon as they’re sent to the Checkout page, the order is final and they can’t modify it without creating a new Checkout Session.
Run the application
Start your server and navigate to http://localhost:3000/checkout
ruby server.rb
Try it out
Click the checkout button to be redirected to the Stripe Checkout page. Use any of these test cards to simulate a payment.
Congratulations!
You have a basic Checkout integration working. Now learn how to customize the appearance of your checkout page and automate tax collection.
Customize the appearance of the hosted Checkout page by:
- Adding your logo and color theme in your branding settings.
- Using the Checkout Sessions API to activate additional features, like collecting addresses or prefilling customer data.
Calculate and collect the right amount of tax on your Stripe transactions. Learn more about Stripe Tax and how to add it to Checkout.
Next steps
Fulfill orders
Set up an event destination to fulfill orders after a payment succeeds and to handle other critical events.
Receive payouts
Learn how to move funds out of your Stripe account into your bank account.
Refund and cancel payments
Handle requests for refunds by using the Stripe API or Dashboard.
Customer management
Let your customers self-manage their payment details, invoices, and subscriptions.
Adaptive Pricing
Automatically present prices in your customer’s local currency.
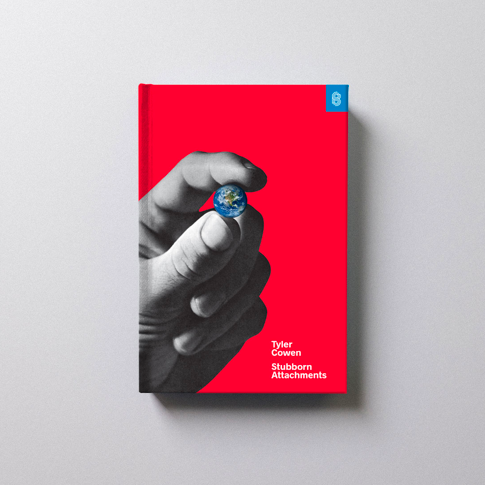
Stubborn Attachments
$20.00
require 'stripe'require 'sinatra'# This test secret API key is a placeholder. Don't include personal details in requests with this key.# To see your test secret API key embedded in code samples, sign in to your Stripe account.# You can also find your test secret API key at https://dashboard.stripe.com/test/apikeys.Stripe.api_key = 'sk_test_BQokikJOvBiI2HlWgH4olfQ2'set :static, trueset :port, 4242YOUR_DOMAIN = 'http://localhost:4242'post '/create-checkout-session' docontent_type 'application/json'session = Stripe::Checkout::Session.create({line_items: [{# Provide the exact Price ID (e.g. pr_1234) of the product you want to sellprice: '{{PRICE_ID}}',quantity: 1,}],mode: 'payment',success_url: YOUR_DOMAIN + '?success=true',cancel_url: YOUR_DOMAIN + '?canceled=true',})redirect session.url, 303end
source 'https://rubygems.org/'gem 'sinatra'gem 'stripe'
{"name": "client","version": "0.1.0","private": true,"dependencies": {"@stripe/react-stripe-js": "^1.0.0","@stripe/stripe-js": "^1.0.0","react": "^16.9.0","react-dom": "^16.9.0","react-scripts": "^3.4.0"},"homepage": "http://localhost:3000/checkout","proxy": "http://localhost:4242","scripts": {"start": "react-scripts start","build": "react-scripts build","test": "react-scripts test","eject": "react-scripts eject"},"eslintConfig": {"extends": "react-app"},"browserslist": {"production": [">0.2%","not dead","not op_mini all"],"development": ["last 1 chrome version","last 1 firefox version","last 1 safari version"]}}
import React, { useState, useEffect } from "react";import "./App.css";const ProductDisplay = () => (<section><div className="product"><imgsrc="https://i.imgur.com/EHyR2nP.png"alt="The cover of Stubborn Attachments"/><div className="description"><h3>Stubborn Attachments</h3><h5>$20.00</h5></div></div><form action="/create-checkout-session" method="POST"><button type="submit">Checkout</button></form></section>);const Message = ({ message }) => (<section><p>{message}</p></section>);export default function App() {const [message, setMessage] = useState("");useEffect(() => {// Check to see if this is a redirect back from Checkoutconst query = new URLSearchParams(window.location.search);if (query.get("success")) {setMessage("Order placed! You will receive an email confirmation.");}if (query.get("canceled")) {setMessage("Order canceled -- continue to shop around and checkout when you're ready.");}}, []);return message ? (<Message message={message} />) : (<ProductDisplay />);}
body {display: flex;justify-content: center;align-items: center;background: #242d60;font-family: -apple-system, BlinkMacSystemFont, 'Segoe UI', 'Roboto','Helvetica Neue', 'Ubuntu', sans-serif;height: 100vh;margin: 0;-webkit-font-smoothing: antialiased;-moz-osx-font-smoothing: grayscale;}section {background: #ffffff;display: flex;flex-direction: column;width: 400px;height: 112px;border-radius: 6px;justify-content: space-between;}.product {display: flex;}.description {display: flex;flex-direction: column;justify-content: center;}p {font-style: normal;font-weight: 500;font-size: 14px;line-height: 20px;letter-spacing: -0.154px;color: #242d60;height: 100%;width: 100%;padding: 0 20px;display: flex;align-items: center;justify-content: center;box-sizing: border-box;}img {border-radius: 6px;margin: 10px;width: 54px;height: 57px;}h3,h5 {font-style: normal;font-weight: 500;font-size: 14px;line-height: 20px;letter-spacing: -0.154px;color: #242d60;margin: 0;}h5 {opacity: 0.5;}button {height: 36px;background: #556cd6;color: white;width: 100%;font-size: 14px;border: 0;font-weight: 500;cursor: pointer;letter-spacing: 0.6;border-radius: 0 0 6px 6px;transition: all 0.2s ease;box-shadow: 0px 4px 5.5px 0px rgba(0, 0, 0, 0.07);}button:hover {opacity: 0.8;}
import React from "react";import ReactDOM from "react-dom";import App from "./App";ReactDOM.render(<App />, document.getElementById("root"));
<!DOCTYPE html><html lang="en"><head><meta charset="utf-8" /><link rel="shortcut icon" href="%PUBLIC_URL%/favicon.ico" /><meta name="viewport" content="width=device-width, initial-scale=1" /><meta name="theme-color" content="#000000" /><meta name="description" content="A demo of a payment on Stripe" /><title>Stripe sample</title></head><body><noscript>You need to enable JavaScript to run this app.</noscript><div id="root"></div><!--This HTML file is a template.If you open it directly in the browser, you will see an empty page.You can add webfonts, meta tags, or analytics to this file.The build step will place the bundled scripts into the <body> tag.To begin the development, run `npm start` or `yarn start`.To create a production bundle, use `npm run build` or `yarn build`.--></body></html>
# Accept a Payment with Stripe CheckoutStripe Checkout is the fastest way to get started with payments. Included are some basic build and run scripts you can use to start up the application.## Set Price IDIn the back end code, replace `{{PRICE_ID}}` with a Price ID (`price_xxx`) that you created.## Running the sample1. Build the server~~~bundle install~~~2. Run the server~~~ruby server.rb -o 0.0.0.0~~~3. Build the client app~~~npm install~~~4. Run the client app~~~npm start~~~5. Go to [http://localhost:3000/checkout](http://localhost:3000/checkout)